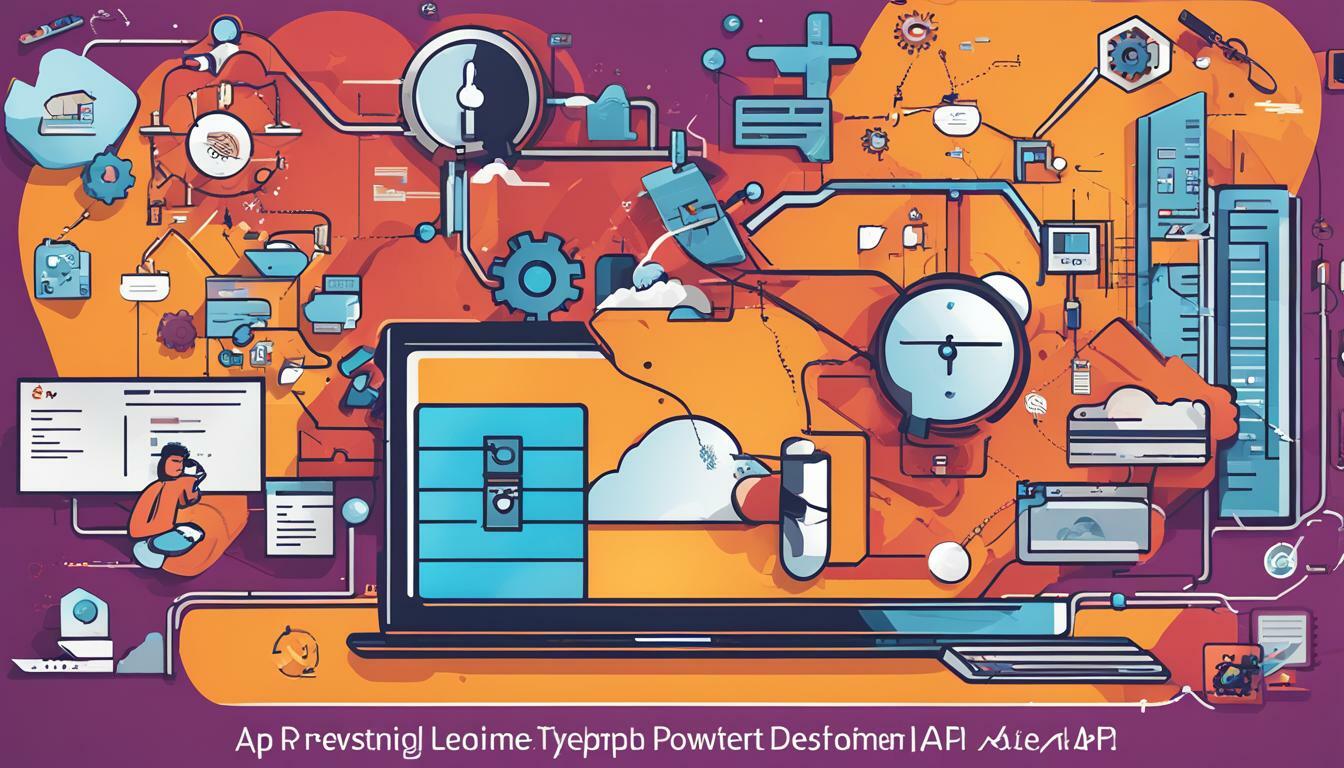
As a .NET developer, you understand the importance of creating a reliable and efficient API. However, the ability to enhance your .NET API with proven strategies and examples is what differentiates a successful API from an average one. In this article, we will explore the best practices, techniques, and examples that will enable you to develop an efficient and successful .NET API.
From .NET API enhancement strategies to .NET API examples, we will dive into the fundamental concepts and advanced techniques for API enhancement. We will also discuss the importance of enhancing the usability, security, and performance of your .NET API, as well as the significance of creating comprehensive API documentation.
By the end of this article, you will have the necessary tools and knowledge to unlock the full potential of your .NET API. Let’s get started!
Understanding the Basics of .NET API Enhancement
Welcome to our guide on enhancing your .NET API. To accomplish this, you need to employ strategies for improving its performance, optimizing its functionality, and ensuring its efficient usage. In this section, we’ll discuss the proven techniques and optimization strategies you can implement to enhance your .NET API.
Strategies for Enhancing .NET API
Improving your .NET API involves a range of strategies that can help unlock its full potential. Some of the most effective strategies include:
- Optimizing API responses for faster performance.
- Implementing caching mechanisms to reduce database calls.
- Introducing API versioning for better management.
- Employing asynchronous programming to handle large data payloads.
By following these strategies, you can significantly improve your .NET API’s performance and ensure efficient usage.
Proven Techniques for Improving .NET API Performance
Improving your .NET API performance is a multifaceted process that requires a range of techniques. Some of the most reliable techniques include:
- Employing response compression to reduce data transfer times.
- Reducing data transfer times by using binary serialization.
- Optimizing database connections for faster data retrieval.
- Employing load balancing to distribute client requests across multiple servers.
By implementing these techniques, you can significantly improve your .NET API’s performance and ensure its reliability.
Optimizing .NET API
Optimizing your .NET API involves a range of practices that can help ensure its efficient usage. Some of the most effective practices include:
- Minimizing the number of database calls.
- Developing an efficient API architecture that promotes scalability.
- Employing efficient error handling mechanisms.
- Using RESTful API endpoints for better resource utilization.
By optimizing your .NET API with these practices, you can ensure its efficiency and promote its scalability.
Pro Tip: Keep in mind that enhancing your .NET API is an ongoing process. Continuously monitoring your API’s performance and making necessary improvements will help you stay ahead of the curve and ensure its long-term success.
Advanced Techniques for .NET API Enhancement
Developing an efficient API architecture is crucial to the success of your .NET API. The first step is to identify the purpose and functionality of your API. This will help you determine the appropriate architecture and design pattern to implement. Some of the popular design patterns used in .NET API are:
- Model-View-Controller (MVC)
- Repository Pattern
- Service Layer Pattern
- Decorator Pattern
Implementing successful API strategies involves understanding the intended audience of your API and their requirements. You should design your API in a way that is easy to use and understand, and make sure it meets the needs of your audience. Some of the strategies for improving API usage are:
- Versioning your API to manage changes
- Implementing caching mechanisms to improve performance
- Providing clear and concise error messages to assist with debugging
- Using appropriate HTTP status codes to indicate the outcome of operations
Leveraging advanced .NET API techniques can help you accomplish specific goals and enhance the functionality of your API. Some of the advanced techniques include:
- Implementing asynchronous programming to improve performance
- Using dependency injection to reduce coupling and improve maintainability
- Implementing custom middleware to add additional functionality to your API
- Using OpenAPI (formerly known as Swagger) to document your API and provide an interactive API console
By implementing these advanced techniques, you can take your .NET API to the next level and provide a better experience for your users.
Best Practices for Enhancing .NET API Usability
Enhancing the usability and functionality of your .NET API is crucial for providing a positive user experience. By following best practices for API development, you can ensure that your API is intuitive, well-documented, and easy to use.
Designing Intuitive API Interfaces
The first step in enhancing the usability of your .NET API is to design an intuitive API interface. This involves using clear and concise naming conventions for API endpoints, utilizing HTTP methods appropriately, and organizing endpoints into logical groups.
When designing your API, consider the needs of your users and strive to make the API interface as user-friendly as possible. This can be achieved by following established API design principles and consulting API usability guidelines.
Providing Comprehensive Documentation
Comprehensive documentation is essential for enhancing the usability of your .NET API. Documentation should provide an overview of the API, including its purpose, endpoints, and required parameters.
In addition, documentation should include code samples, along with guidelines for using the API effectively. This will help users to quickly understand how to use your API and reduce the time required to integrate with it.
Implementing Error Handling Mechanisms
Error handling is a critical aspect of API development. By implementing proper error handling mechanisms, you can ensure that your API is robust, reliable, and easy to use.
When designing error handling logic, consider the potential errors that users may encounter and provide clear, concise error messages that are easy to understand. In addition, consider implementing error codes and status codes to help users quickly identify and resolve errors.
By following these best practices for enhancing the usability and functionality of your .NET API, you can develop an API that provides a positive user experience and facilitates seamless integration with other applications.
Improving .NET API Security and Performance
Ensuring the security and performance of your .NET API is crucial to its success. To achieve this goal, there are several strategies and proven techniques that can be implemented.
Implementing Authentication and Authorization Mechanisms
One way to enhance the security of your .NET API is by implementing authentication and authorization mechanisms. This involves verifying the identity of the user accessing the API and ensuring they have permission to access specific resources. By doing so, you can protect sensitive data and prevent unauthorized access to your API.
Optimizing API Responses
Optimizing your API responses is another effective way to improve its performance. By minifying and compressing responses, you can reduce the amount of data that needs to be transferred over the network, leading to faster response times. Additionally, implementing caching mechanisms can help reduce the load on the server and improve overall API performance.
Handling Large Data Payloads
If your API deals with large amounts of data, handling it efficiently can be a challenge. To address this, you can implement techniques such as pagination, filtering, and sorting to reduce the amount of data returned in each request. Additionally, employing compression and chunking can help optimize data transfer and improve performance.
By implementing these strategies and techniques, you can enhance the security and performance of your .NET API, ensuring its success and reliability.
Harnessing the Power of API Documentation
Documentation is a crucial component of enhancing the usability and functionality of your .NET API. Your API’s documentation should be user-friendly, comprehensive, and up-to-date to enable users to implement your API seamlessly. Below are some best practices for creating effective .NET API documentation:
- Use simple language: Your documentation should be easy to read and understand, even for non-technical users. Avoid using jargon and complex terms.
- Provide clear examples: Examples are a great way to help users understand the API’s functionality. They should be concise and easy to understand.
- Use proper formatting: Use formatting to make your documentation easy to scan, with headings, bullets, and other formatting tools used to structure your content.
- Provide comprehensive documentation: Include detailed information about each endpoint, such as parameters, responses, and expected errors.
- Provide references: Include links to any additional resources that may be necessary for users to understand and implement your API.
Using .NET API documentation tools such as Swagger, you can easily generate documentation that adheres to these best practices. By providing clear and comprehensive documentation, you make it easier for users to understand and implement your API, leading to enhanced usability and functionality.
Below are some examples of .NET API documentation that effectively adheres to these best practices:
“POST /user/register: Register a new user account with the provided details”
Parameters:
- Username: string (required)
- Password: string (required)
- Email: string (required)
Response:
- Status: 200 OK
- Message: “User account created successfully”
By following these best practices, you can ensure that your .NET API documentation is clear, concise, and user-friendly, leading to enhanced usability and functionality.
Testing and Debugging Your .NET API
To ensure the reliability and functionality of your .NET API, it’s essential to perform thorough testing and debugging. There are several testing methodologies, tools, and frameworks available that can assist in this process.
Unit testing is a popular testing methodology where individual units or components of an application are tested to verify that they perform as expected. With integration testing, multiple units are tested together to verify that they work as expected when integrated. Functional testing tests the functionality of a system as a whole, and performance testing aims to identify performance bottlenecks that may affect the API’s performance.
Debugging is crucial to find and fix errors or defects in code. In .NET API, Visual Studio Debugger is a popular tool used for debugging. It allows developers to step through code and examine variables and objects at runtime.
When testing and debugging your .NET API, it’s essential to use test data that accurately represents the type and volume of data that the API will handle in production. This is where mocking frameworks come in. They allow developers to create mock objects and test the API’s behavior without relying on real data.
Real-world .NET API examples can help illustrate the testing and debugging process. For instance, when testing a weather API, you could create test data to simulate different weather conditions and then use integration testing to verify that the API returns the expected results for each condition.
Conclusion
Enhancing your .NET API with proven strategies and techniques is key to unlocking its full potential. Throughout this article, we have explored the fundamental concepts of .NET API enhancement and discussed advanced techniques for improving its performance, usability, and security.
We have covered best practices for designing intuitive API interfaces, providing comprehensive documentation, and implementing effective error handling mechanisms. We also delved into the importance of testing and debugging in the development of a robust .NET API.
By implementing these strategies and techniques, you can take your .NET API to new heights of efficiency, security, and functionality. Remember to stay up-to-date with the latest advancements in .NET API development to stay ahead of the competition.
Take Action Today
Don’t wait any longer to optimize your .NET API for success. Implement the strategies and techniques discussed in this article to unlock its full potential. With the right approach, you can develop an API that users will love and that will deliver outstanding results for your business.